Are you tired of wrestling with API bottlenecks and scalability issues in your Laravel projects? 🤔 As your application grows, so does the challenge of maintaining a robust and efficient API. Building scalable APIs is not just a luxury—it’s a necessity in today’s fast-paced digital landscape.
Laravel, known for its elegance and simplicity, offers powerful tools for API development. But without the right approach, even the most promising Laravel APIs can crumble under heavy loads. That’s where mastering best practices and insider tips comes into play. Whether you’re battling slow response times, grappling with authentication complexities, or simply aiming to future-proof your API, this guide is your roadmap to success.
In this comprehensive blog post, we’ll dive deep into the world of scalable API development with Laravel. From understanding the framework’s API architecture to implementing advanced performance optimization techniques, we’ll cover everything you need to know. Get ready to transform your Laravel APIs from good to great, as we explore key topics like designing efficient endpoints, optimizing database interactions, and mastering authentication and authorization. Let’s embark on this journey to API excellence together! 💪🚀
Table of Contents
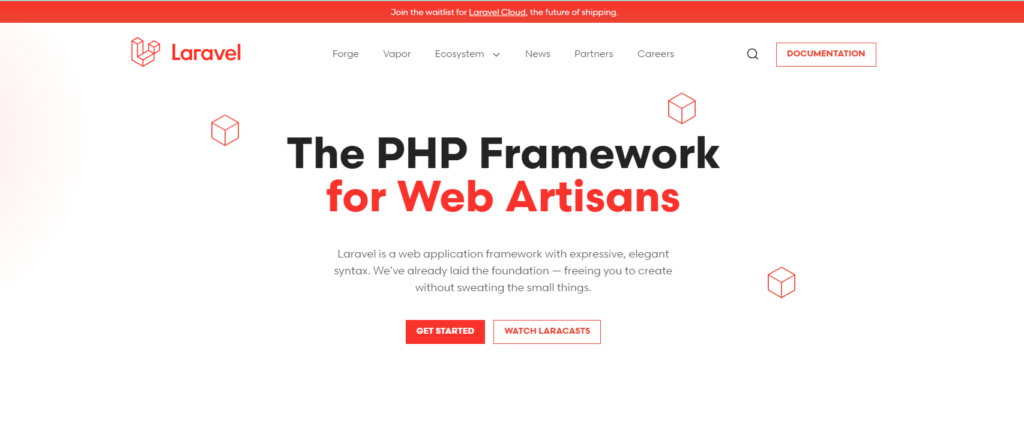
Understanding Laravel’s API Architecture
Key components of Laravel’s API structure
Laravel provides a robust foundation for building scalable APIs. The key components include:
- Routes: Define API endpoints using Laravel’s routing system
- Controllers: Handle API requests and return responses
- Middleware: Process requests before they reach the controller
- Models: Represent database tables and handle data interactions
- Resources: Transform data into JSON responses
RESTful API principles in Laravel
Laravel makes it easy to adhere to RESTful principles:
- Use HTTP methods (GET, POST, PUT, DELETE) appropriately
- Implement resource-based URLs
- Return proper status codes
- Use JSON for data exchange
Benefits of using Laravel for API development
Benefit | Description |
---|---|
Rapid Development | Built-in tools and features accelerate API creation |
Security | Robust security features protect against common vulnerabilities |
Scalability | Efficient request handling and caching mechanisms |
Flexibility | Easy integration with various front-end frameworks |
Laravel’s architecture provides a solid foundation for building scalable and maintainable APIs. Its adherence to RESTful principles ensures consistency and interoperability. With these benefits in mind, let’s explore how to design scalable API endpoints in Laravel.
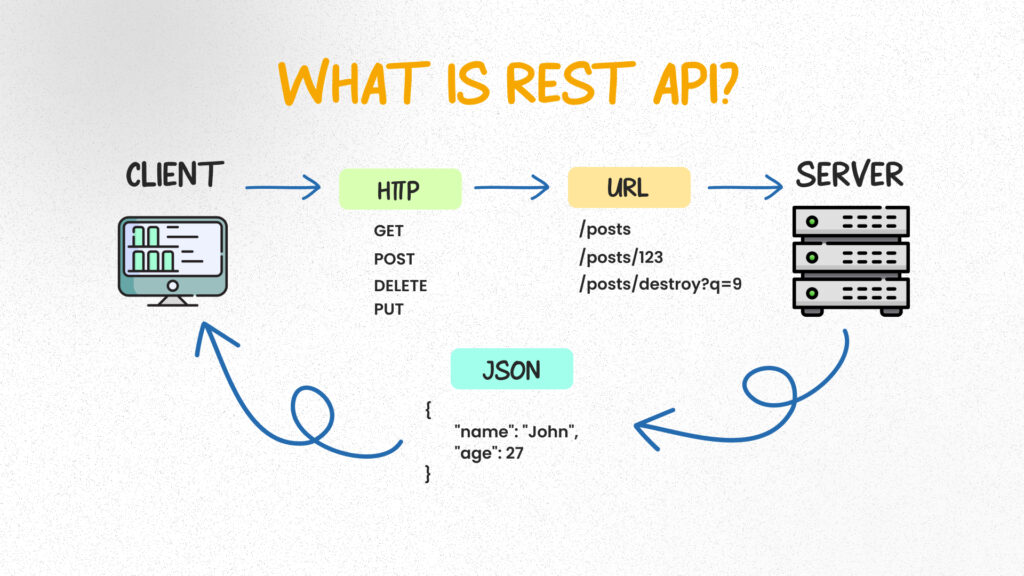
Designing Scalable API Endpoints
When building APIs with Laravel, designing scalable endpoints is crucial for long-term success. Let’s explore key strategies to ensure your API can handle growth and maintain performance.
A. Handling pagination for large datasets
Pagination is essential for managing large datasets efficiently. Laravel provides built-in pagination support, making it easy to implement:
$users = User::paginate(20);
This approach prevents overwhelming responses and improves performance. Consider implementing:
- Cursor-based pagination for real-time data
- Offset-based pagination for static data
B. Versioning your API
API versioning allows you to make changes without breaking existing client integrations. Laravel supports multiple versioning strategies:
- URL versioning:
/api/v1/users
- Header versioning:
Accept: application/vnd.myapp.v1+json
- Query parameter:
/api/users?version=1
Choose a method that aligns with your project’s needs and stick to it consistently.
C. Implementing proper HTTP methods
Utilize HTTP methods correctly to create a RESTful API:
HTTP Method | Usage |
---|---|
GET | Retrieve resources |
POST | Create new resources |
PUT/PATCH | Update existing resources |
DELETE | Remove resources |
Implementing these methods correctly improves API clarity and adherence to RESTful principles.
D. Creating meaningful and consistent URL structures
Design clear and consistent URL structures to enhance API usability:
- Use nouns for resources:
/api/articles
instead of/api/getArticles
- Nest related resources:
/api/articles/{id}/comments
- Use query parameters for filtering:
/api/articles?category=technology
Consistent URL structures make your API more intuitive and easier to use for developers.
By implementing these best practices, you’ll create a scalable API that can grow with your application’s needs. Next, we’ll explore how to optimize database interactions to further enhance your API’s performance.
Optimizing Database Interactions
As we delve deeper into building scalable APIs with Laravel, let’s focus on optimizing database interactions, a crucial aspect of enhancing API performance.
A. Utilizing caching strategies
Caching is a powerful tool for reducing database load and improving response times. Laravel offers robust caching mechanisms that can be easily implemented:
- Query caching: Store frequently accessed data in cache to minimize database queries.
- Full-page caching: Cache entire API responses for static or semi-static content.
- Cache tags: Group related cached items for efficient cache management.
B. Leveraging Laravel’s query builder
Laravel’s query builder provides a fluent interface for constructing database queries:
| Method | Description |
|-----------------------|---------------------------------------------------|
| `select()` | Specify columns to retrieve |
| `where()` | Add conditions to the query |
| `orderBy()` | Sort the results |
| `groupBy()` | Group the query results |
| `join()` | Perform table joins |
Using the query builder can lead to more efficient and optimized queries compared to raw SQL statements.
C. Implementing database indexing
Proper indexing is crucial for fast data retrieval:
- Identify frequently queried columns
- Create appropriate indexes on these columns
- Use composite indexes for multi-column queries
- Regularly analyze and optimize indexes
D. Efficient use of Eloquent ORM
While Eloquent ORM provides convenience, it’s important to use it efficiently:
- Eager loading: Use
with()
to load related models in a single query - Chunking: Process large datasets in smaller chunks to conserve memory
- Selecting specific columns: Avoid
select *
and retrieve only necessary data
By implementing these optimization techniques, you’ll significantly improve your API’s database interactions, leading to better performance and scalability.
Implementing Authentication and Authorization
Now that we’ve optimized our database interactions, let’s focus on securing our API. Authentication and authorization are crucial components of any scalable API, ensuring that only authorized users can access specific endpoints and perform certain actions.
Securing API routes
Securing API routes is the first step in implementing authentication. Laravel provides middleware that can be easily applied to routes or route groups:
Route::middleware('auth:api')->group(function () {
Route::get('/user', 'UserController@show');
Route::post('/order', 'OrderController@store');
});
This middleware ensures that only authenticated users can access these routes.
Implementing role-based access control
Role-based access control (RBAC) adds an extra layer of security by restricting access based on user roles:
Role | Permissions |
---|---|
Admin | Full access |
Manager | Read/Write access |
User | Read-only access |
To implement RBAC, you can create custom middleware or use Laravel’s built-in Gate facade:
Gate::define('update-post', function ($user, $post) {
return $user->role === 'admin' || $user->id === $post->user_id;
});
Using JSON Web Tokens (JWT)
JSON Web Tokens provide a secure way to transmit information between parties as a JSON object. They’re particularly useful for stateless authentication in APIs:
- User logs in with credentials
- Server verifies and returns a JWT
- Client includes JWT in subsequent requests
- Server validates JWT for each request
Setting up Laravel Passport
Laravel Passport is an OAuth2 server implementation that makes API authentication a breeze:
- Install Passport via Composer
- Run migrations and install Passport
- Add the Laravel\Passport\HasApiTokens trait to your User model
- Register Passport routes in AuthServiceProvider
With Passport set up, you can easily issue access tokens and secure your API endpoints.
As we move forward, we’ll explore how to handle errors and implement proper logging in our Laravel API.
Error Handling and Logging
Now that we’ve covered authentication and authorization, let’s dive into the crucial aspects of error handling and logging in Laravel APIs.
Monitoring API Performance
Effective monitoring is essential for maintaining a high-performing API. Here are some key practices:
- Use Laravel Telescope for local development monitoring
- Implement New Relic or Datadog for production environments
- Set up custom metrics to track important KPIs
Tool | Local Development | Production | Features |
---|---|---|---|
Laravel Telescope | ✅ | ❌ | Request monitoring, database queries |
New Relic | ❌ | ✅ | Performance metrics, error tracking |
Datadog | ❌ | ✅ | Custom metrics, log management |
Effective Logging Practices
Proper logging helps in debugging and understanding API behavior:
- Use Laravel’s built-in logging facade
- Implement log levels appropriately (debug, info, warning, error)
- Include relevant context in log messages
- Rotate logs to prevent disk space issues
Implementing Consistent Error Responses
Consistency in error responses improves API usability:
- Create a standardized error response format
- Use appropriate HTTP status codes
- Include error codes and messages in the response body
Example error response structure:
{
"error": {
"code": "VALIDATION_ERROR",
"message": "The given data was invalid.",
"details": [
{
"field": "email",
"message": "The email field is required."
}
]
}
}
Creating Custom Exception Handlers
Custom exception handlers allow for fine-grained control over error responses:
- Extend the base Exception Handler class
- Create specific exception classes for different error types
- Implement render methods to format the response
By implementing these error handling and logging practices, you’ll be better equipped to maintain and troubleshoot your Laravel API. Next, we’ll explore the importance of testing and documentation in ensuring API reliability and usability.
Testing and Documentation
As we delve into the crucial aspects of API development, let’s explore the importance of testing and documentation in building robust and maintainable Laravel APIs.
A. Implementing continuous integration for API development
Continuous Integration (CI) is essential for maintaining code quality and ensuring smooth collaboration. Here’s how to implement CI for your Laravel API:
- Choose a CI tool (e.g., Jenkins, Travis CI, or GitLab CI)
- Set up automated builds and tests
- Configure notifications for failed builds
- Integrate with version control systems
B. Generating API documentation with tools like Swagger
Clear and comprehensive documentation is vital for API adoption. Swagger (OpenAPI) is an excellent tool for generating interactive API documentation:
- Install the
darkaonline/l5-swagger
package - Annotate your API controllers and models
- Generate and publish Swagger documentation
- Keep documentation up-to-date with code changes
C. Automated API testing with Laravel Dusk
Laravel Dusk provides a powerful framework for browser automation and testing:
- Install Laravel Dusk
- Write browser tests for API endpoints
- Simulate user interactions and API calls
- Run tests in a headless browser for CI integration
D. Writing unit and integration tests
Comprehensive testing ensures API reliability and stability:
Test Type | Purpose | Examples |
---|---|---|
Unit Tests | Test individual components | Model methods, Helper functions |
Integration Tests | Test interactions between components | API endpoints, Database operations |
Feature Tests | Test complete features | User registration, Data retrieval |
To implement effective testing:
- Use Laravel’s built-in testing tools
- Write tests for each API endpoint
- Cover both successful and error scenarios
- Maintain a high test coverage percentage
By focusing on thorough testing and clear documentation, you’ll create a more maintainable and developer-friendly API. Next, we’ll explore performance optimization techniques to ensure your Laravel API can handle high traffic and scale effectively.
Performance Optimization Techniques
As we delve into performance optimization techniques, we’ll explore various strategies to enhance the speed and efficiency of your Laravel API.
Leveraging Laravel Horizon for Queue Management
Laravel Horizon provides a beautiful dashboard and code-driven configuration for your Redis queues. It allows you to easily monitor key metrics of your queue system in real-time, including job throughput, runtime, and job failures.
- Easily configure and manage multiple queue workers
- Monitor queue performance in real-time
- Customize queue worker settings for optimal performance
Implementing Asynchronous Processing
For time-consuming tasks, asynchronous processing can significantly improve API response times:
- Identify resource-intensive operations
- Move these operations to background jobs
- Use Laravel’s queue system to manage these jobs
Optimizing Database Queries
Efficient database interactions are crucial for API performance. Here are some techniques:
- Use eager loading to avoid N+1 query problems
- Implement database indexing for frequently queried columns
- Utilize query caching for repetitive queries
Utilizing Laravel’s Built-in Caching Mechanisms
Laravel offers robust caching support out of the box. Here’s how you can leverage it:
Caching Technique | Use Case |
---|---|
Route caching | Speeds up route registration |
Config caching | Combines all config files into a single file |
View caching | Pre-compiles Blade templates |
Data caching | Stores query results or API responses |
Implementing API Rate Limiting
Rate limiting helps prevent abuse and ensures fair usage of your API:
- Use Laravel’s built-in rate limiting middleware
- Customize rate limits based on user roles or API endpoints
- Implement exponential backoff for repeated violations
By implementing these performance optimization techniques, you can significantly enhance the scalability and responsiveness of your Laravel API. Next, we’ll recap the key points discussed in this blog post and provide some final thoughts on building scalable APIs with Laravel.
Conclusion
Building scalable APIs with Laravel requires a comprehensive approach that encompasses design, implementation, and optimization. By understanding Laravel’s API architecture, designing efficient endpoints, and optimizing database interactions, developers can create robust and high-performance APIs. Implementing proper authentication, authorization, and error handling ensures security and reliability, while thorough testing and documentation contribute to maintainability and ease of use.
To create truly scalable APIs, it’s crucial to focus on performance optimization techniques. By leveraging Laravel’s built-in features and following best practices, developers can build APIs that not only meet current needs but also scale effortlessly to accommodate future growth. Remember, scalability is an ongoing process, so continuously monitor, test, and refine your API to ensure it remains efficient and responsive as your user base expands.