Laravel 11 has officially launched, introducing several exciting new features, including a minimum PHP version requirement of 8.2, a brand-new Laravel Reverb package, a more streamlined directory structure, and much more. Let’s dive into what’s new in this release!
# Laravel Reverb
Laravel Reverb is a newly introduced first-party WebSocket server that enhances real-time communication between the client and server in Laravel applications. Some of its standout features include:
Blazing Fast
Reverb is engineered for high performance. A single server can manage thousands of simultaneous connections, transmitting data without the inefficiencies and delays commonly associated with HTTP polling.
Seamless Integration
Reverb integrates smoothly with Laravel’s broadcasting features. You can easily deploy Reverb through Laravel Forge and monitor it via Pulse’s built-in support.
Built for Scale
Thanks to Reverb’s built-in horizontal scaling with Redis, you can expand your capacity as needed, managing connections and channels across multiple servers.
Pusher Compatibility
Reverb uses the Pusher protocol for WebSockets, making it fully compatible with Laravel broadcasting and Laravel Echo from day one.
# Streamlined Directory Structure
A fresh installation of Laravel 11 comes with a significant reduction in files—approximately 69 fewer files. Nice!
For more details, check out our post on the revamped Laravel directory structure.
- Controllers: No longer extend anything by default.
- Middleware Directory: The middleware directory has been removed. Laravel still includes nine middleware by default, though many are rarely customized. If you need to, customization now happens in the
App/ServiceProvider
:
public function boot(): void
{
EncryptCookies::except(['some_cookie']);
}
# No More Http/Kernel
Most of the functionality previously handled by the Kernel can now be accomplished in the Bootstrap/App file:
return Application::configure()
->withProviders()
->withRouting(
web: __DIR__.'/../routes/web.php',
commands: __DIR__.'/../routes/console.php',
)
->withMiddleware(function(Middleware $middleware) {
$middleware->web(append: LaraconMiddleware::class);
});
# Model Casts Changes
In Laravel 11, model casts are now defined as methods rather than properties. This new approach allows you to do more, such as calling methods directly from the casts. Here’s an example with the new AsEnumCollection
:
protected function casts(): array
{
return [
'email_verified_at' => 'datetime',
'password' => 'hashed',
'options' => AsEnumCollection::of(UserOption::class),
];
}
# New Dumpable Trait
To simplify the framework, Laravel 11 introduces the Dumpable
trait, replacing multiple “dd” or “dump” methods across different classes. You can also use this trait in your own classes:
class Stringable implements JsonSerializable, ArrayAccess
{
use Conditionable, Dumpable, Macroable, Tappable;
str('foo')->dd();
str('foo')->dump();
}
# Config Changes
Laravel 11 streamlines the configuration files, with many config options cascading down. The .env
file now includes expanded options to cover most of your configuration needs.
# New Once Method
The new once
helper ensures that a method always returns the same value, regardless of how many times it is called. This is particularly useful when you want certain code to run only once during the lifecycle of an application.
# Slimmed Default Migrations
In this version, the default migrations, which previously spanned from 2014 to 2019, have been condensed into just two files. The dates are now removed.
# Routes Changes
By default, Laravel 11 will now only include two route files: console.php
and web.php
. API routes are opt-in, and you can install them via the php artisan install:api
command. Similarly, for WebSocket broadcasting, use php artisan install:broadcasting
.
# New /up Health Route
Laravel 11 introduces a new /up
health route, which fires a DiagnosingHealthEvent
. This route helps with uptime monitoring and provides better insights into the health of your application.
# APP_KEY Rotation
In previous versions of Laravel, changing your APP_KEY
could cause issues with encrypted data. Laravel 11 introduces graceful rotation, allowing the key to be changed without breaking any old encrypted data. The new APP_PREVIOUS_KEYS
environment variable allows for seamless key rotation.
# Console Kernel Removed
The Console Kernel is no longer part of Laravel 11. Instead, you can define your console commands directly in routes/console.php
.
# Named Arguments
Named arguments are now allowed in Laravel 11, but they aren’t covered under Laravel’s backward compatibility guidelines. Be cautious when using them, as parameter names may change in future Laravel versions.
# Eager Load Limit
Laravel 11 incorporates the “eager load limit” package, letting you limit eager-loaded relationships directly within your queries:
User::select('id', 'name')->with([
'articles' => fn($query) => $query->limit(5)
])->get();
# New Artisan Commands
Several new Artisan commands are now available in Laravel 11 to quickly create classes, enums, interfaces, and traits:
php artisan make:class
php artisan make:enum
php artisan make:interface
php artisan make:trait
# New Welcome Page
Laravel 11 introduces a fresh new welcome page when creating a new Laravel application. This page comes with a modern design and useful information about Laravel.
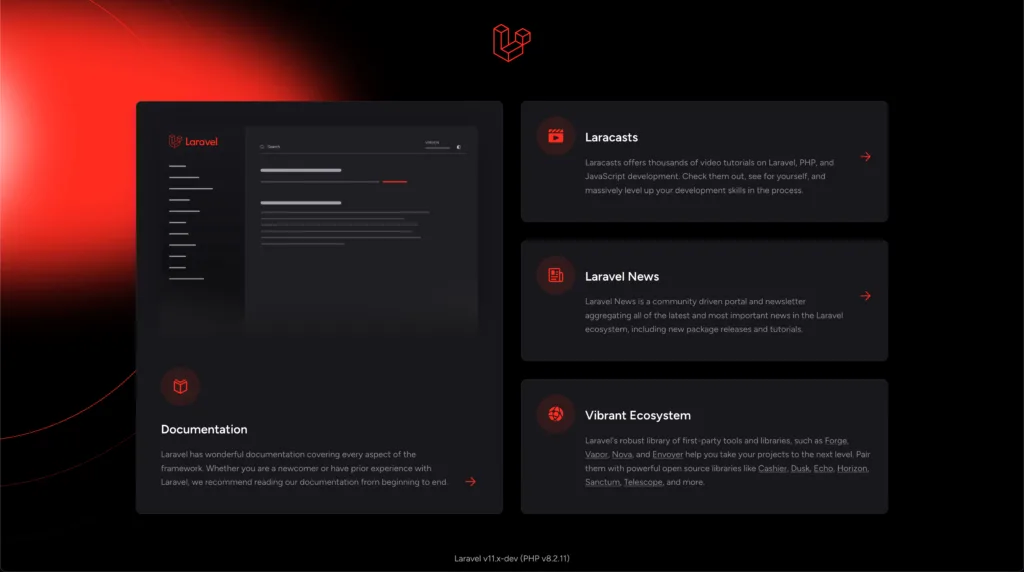
# When Will Laravel 11 Be Released?
Laravel 11 will officially be released on March 12, 2024.
# PHP 8.2 Minimum Support
Laravel 11 requires a minimum of PHP 8.2 to run. If you’re using an older version of PHP, now is the time to upgrade.
# SQLite 3.35.0+ Required
For users working with SQLite databases, Laravel 11 mandates the use of SQLite version 3.35.0 or higher.
# Doctrine DBAL Removal
Laravel 11 is no longer dependent on the Doctrine DBAL, and custom Doctrine types are no longer necessary for creating or altering column types that previously required custom implementations.
# Install Laravel 11
To install Laravel 11, follow these steps:
composer global require laravel/installer
Then run,
laravel new projectname
# Upgrade to Laravel 11
Laravel Shift offers the easiest way to upgrade your existing project to Laravel 11, though you can also follow the official upgrade guide provided in the Laravel documentation.
# Laravel Support Policy
Laravel provides bug fixes for all releases for 18 months and security fixes for 2 years. Here’s a breakdown of the support policy:
Version | PHP | Release | Bug Fixes Until | Security Fixes Until |
---|---|---|---|---|
Laravel 9 | 8.0 – 8.2 | February 8, 2022 | August 8, 2023 | February 6, 2024 |
Laravel 10 | 8.1 – 8.3 | February 14, 2023 | August 6, 2024 | February 4, 2025 |
Laravel 11 | 8.2 – 8.3 | March 12, 2024 | September 3, 2025 | March 12, 2026 |
Wrap-Up
These are the key features currently announced for Laravel 11, and they’re aimed at enhancing your development workflow. Stay tuned, as more updates will be released, and we’ll keep this post updated with the latest changes!
Explore More on Laravel and PHP
If you’re interested in diving deeper into Laravel and PHP development, check out these related articles on our blog:
- Top 5 PHP Security Practices to Protect Your Applications in 2024
- Laravel Eloquent: Checking for Nested Relationships
These resources provide valuable insights and best practices to enhance your development skills and keep your applications secure. Happy coding!